Card
Generic container for grouping related components together.
#
Documentation
#
#
Usage
#
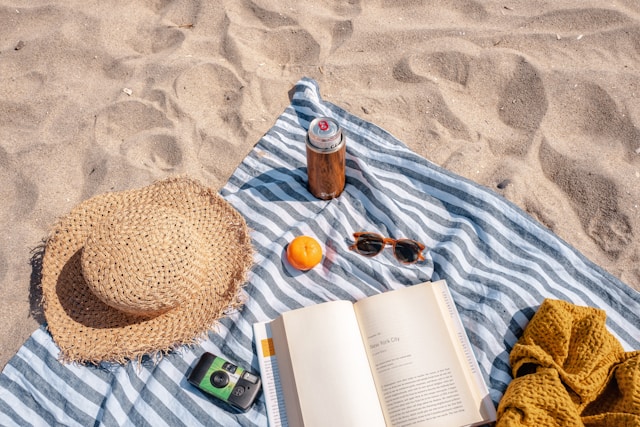
Unveiling the secrets.
import beach from "@/demos/beach.jpg";
import {
DropdownMenu,
DropdownMenuContent,
DropdownMenuItem,
DropdownMenuTrigger,
EllipsisMenuButton,
Flex,
} from "@optiaxiom/react";
import {
Card,
CardAction,
CardContent,
CardDescription,
CardImage,
CardLink,
CardOverflow,
CardTitle,
} from "@optiaxiom/react/unstable";
import { IconLogout, IconPencil } from "@tabler/icons-react";
import Image from "next/image";
export function App() {
return (
<Card maxW="xs">
<CardOverflow>
<CardImage asChild>
<Image
alt="brown glass bottle beside white book on blue and white textile"
priority
src={beach}
/>
</CardImage>
</CardOverflow>
<CardContent>
<Flex flexDirection="row" justifyContent="space-between">
<CardTitle>
<CardLink href="#usage">The majestic world of turtles</CardLink>
</CardTitle>
<CardAction>
<DropdownMenu>
<DropdownMenuTrigger asChild>
<EllipsisMenuButton
appearance="subtle"
aria-label="actions"
size="sm"
/>
</DropdownMenuTrigger>
<DropdownMenuContent align="end">
<DropdownMenuItem icon={<IconPencil />}>Edit</DropdownMenuItem>
<DropdownMenuItem icon={<IconLogout />} intent="danger">
Delete
</DropdownMenuItem>
</DropdownMenuContent>
</DropdownMenu>
</CardAction>
</Flex>
<CardDescription>Unveiling the secrets.</CardDescription>
</CardContent>
</Card>
);
}
#
Anatomy
#
import {
Card,
CardAction,
CardCheckbox,
CardContent,
CardDescription,
CardImage,
CardLink,
CardOverflow,
CardTitle,
} from "@optiaxiom/react/unstable";
export default () => (
<Card>
<CardOverflow>
<CardAction>
<CardCheckbox />
</CardAction>
<CardImage />
</CardOverflow>
<CardImage />
<CardContent>
<CardTitle>
<CardLink />
</CardTitle>
<CardDescription />
<CardAction />
</CardContent>
</Card>
);
#
Props
#
#
Card
#
Supports all Box props in addition to its own. Renders a <div>
element.
Prop |
---|
asChild Change the default rendered element for the one passed as a child, merging their props and behavior. Read the Composition guide for more details.
|
className
|
orientation The orientation/layout of the elements inside the card.
Default: |
#
CardContent
#
Supports all Box props in addition to its own. Renders a <div>
element.
Prop |
---|
asChild Change the default rendered element for the one passed as a child, merging their props and behavior. Read the Composition guide for more details.
|
className
|
#
CardTitle
#
Supports all Box props in addition to its own. Renders a <div>
element.
Prop |
---|
asChild Change the default rendered element for the one passed as a child, merging their props and behavior. Read the Composition guide for more details.
|
className
|
level Switch between the different h1-h6 levels.
|
lineClamp Truncate the text at specific number of lines.
|
truncate Whether to truncate the text and add an ellipsis at the end.
|
#
CardDescription
#
Supports all Box props in addition to its own. Renders a <div>
element.
Prop |
---|
asChild Change the default rendered element for the one passed as a child, merging their props and behavior. Read the Composition guide for more details.
|
className
|
lineClamp Truncate the text at specific number of lines.
|
truncate Whether to truncate the text and add an ellipsis at the end.
|
#
CardImage
#
Supports all Box props in addition to its own. Renders a <div>
element.
Prop |
---|
asChild Change the default rendered element for the one passed as a child, merging their props and behavior. Read the Composition guide for more details.
|
className
|
#
CardOverflow
#
Supports all Box props in addition to its own. Renders a <div>
element.
Prop |
---|
asChild Change the default rendered element for the one passed as a child, merging their props and behavior. Read the Composition guide for more details.
|
className
|
#
CardCheckbox
#
Supports all Box props in addition to its own. Renders a <div>
element.
Prop |
---|
asChild Change the default rendered element for the one passed as a child, merging their props and behavior. Read the Composition guide for more details.
|
className
|
indeterminate Display a partially checked icon instead of the regular checkmark.
|
onCheckedChange
|
#
Changelog
#
#
0.12.1
#
- Added component